Test-Driven Development in SharePoint Framework Projects Use Case
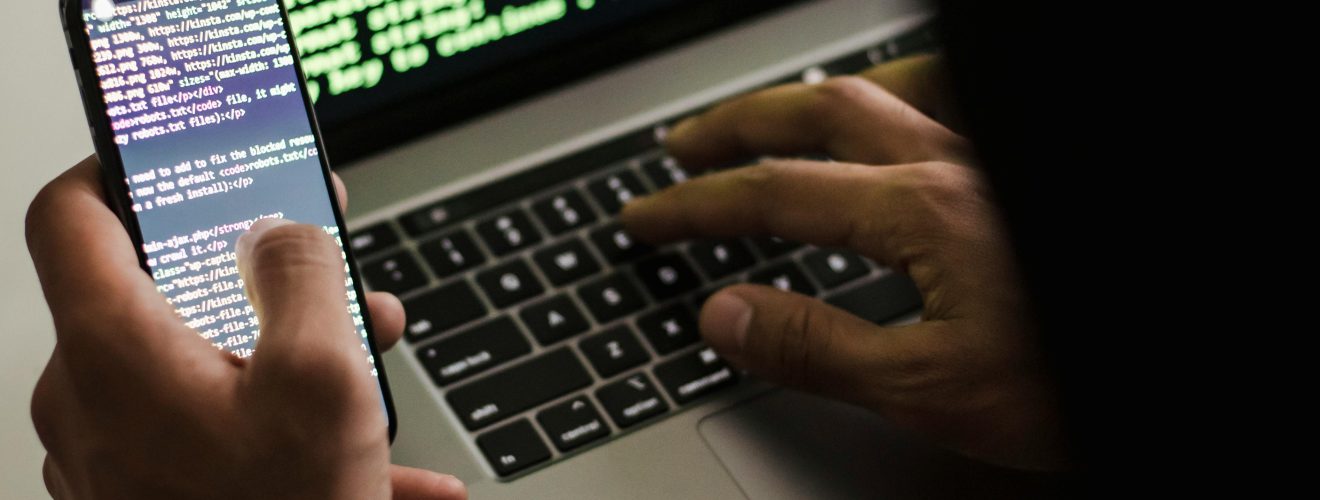
Introduction
In the fast-paced world of software development, ensuring code quality and functionality is paramount. Test-Driven Development (TDD) offers a robust foundation for building reliable SharePoint Framework (SPFx) solutions. By integrating TDD into your SPFx projects, you can enhance code quality, facilitate maintenance, and streamline the development process.
Understanding TDD and SPFx
TDD is a software development approach where tests are written before the actual code. This methodology revolves around a short development cycle: write a test, make it pass, and refactor. SPFx, on the other hand, is a modern design approach for SharePoint development that extends SharePoint’s capabilities to meet complex business needs.
Step-by-Step Guide to TDD with SPFx
- Define the Requirements: Begin by understanding the functional requirements of your SPFx component. What should it accomplish? How will it interact with SharePoint’s features?
- Write the Initial Test: Craft a test that describes the expected behavior of your SPFx component. This test will initially fail, as the feature isn’t implemented yet.
- Implement the Feature: Develop the minimum amount of code required to pass the test. Focus on functionality, not perfection.
- Run and Refine: Execute the test suite. If the test passes, you’ve successfully implemented the feature. If not, refine the code until the test succeeds.
- Refactor and Optimize: With a passing test, optimize your code for readability, structure, and performance without altering its behavior.
- Repeat: Continue this cycle for each new feature or improvement, ensuring a robust and well-tested SPFx solution.
Tools and Frameworks for TDD in SPFx
- Jest: A popular JavaScript testing framework that works well with SPFx components.
- Enzyme: A testing utility for React that makes it easier to assert, manipulate, and traverse your SPFx React components’ output.
- Sinon: Use Sinon for spies, stubs, and mocks in JavaScript.
Mocking SPFx Components
Given that SPFx components are designed to run in the browser, not Node.js, you’ll need to mock SharePoint’s context and other components when running tests. This isolation is crucial for unit testing without the SharePoint environment.
Advanced TDD Strategies for SPFx
- Behavior-Driven Development (BDD): BDD extends TDD by writing tests in a natural language that non-developers can understand, fostering better communication and collaboration.
- Integration Testing: Beyond unit tests, consider how your SPFx components interact with SharePoint and other services.
- Continuous Integration (CI): Automate your testing process by integrating a CI pipeline that runs your tests on every commit, ensuring immediate feedback on the health of your code.
Example 1: Testing a Simple SPFx Web Part
Let’s start with a basic example of a test for an SPFx web part that displays a welcome message.
// WelcomeWebPart.test.ts import { WelcomeWebPart } from './WelcomeWebPart'; import { IWelcomeWebPartProps } from './IWelcomeWebPartProps'; describe('WelcomeWebPart', () => { it('should render a welcome message', () => { // Arrange const props: IWelcomeWebPartProps = { welcomeMessage: 'Hello, SPFx!' }; const webPart = new WelcomeWebPart(props); // Act const message = webPart.render(); // Assert expect(message).toContain('Hello, SPFx!'); }); });
In this test, we’re checking if the WelcomeWebPart
renders the correct welcome message passed through its properties.
Example 2: Testing Asynchronous Operations
Now, let’s look at a more complex example involving asynchronous operations, such as retrieving data from a SharePoint list.
// ListDataFetcher.test.ts import { ListDataFetcher } from './ListDataFetcher'; describe('ListDataFetcher', () => { it('should fetch list items asynchronously', async () => { // Arrange const fetcher = new ListDataFetcher(); const mockItems = [{ Title: 'Test Item 1' }, { Title: 'Test Item 2' }]; fetcher.getListItems = jest.fn().mockResolvedValue(mockItems); // Act const items = await fetcher.getListItems('TestList'); // Assert expect(items.length).toBe(2); expect(items).toEqual(mockItems); }); });
In this test, we’re using Jest’s mocking capabilities to simulate the asynchronous fetching of list items without actually querying a SharePoint list.
Example 3: Testing SPFx Component State Changes
Finally, let’s test a React component’s state changes within an SPFx solution.
// CounterComponent.test.tsx import * as React from 'react'; import { shallow } from 'enzyme'; import { CounterComponent } from './CounterComponent'; describe('CounterComponent', () => { it('should increment the count state', () => { // Arrange const component = shallow(<CounterComponent />); const instance = component.instance() as CounterComponent; // Act instance.incrementCount(); // Assert expect(component.state('count')).toBe(1); }); });
Use Case: Widget Corp’s SPFx Project with TDD

Background: Widget Corp is a mid-sized company specializing in manufacturing and selling widgets. They have a SharePoint online site used by their sales, marketing, and production teams. The company wants to develop a new SPFx web part that allows employees to quickly report and view the status of manufacturing equipment.
Objective: To develop an SPFx web part called “Equipment Status Tracker” that enables employees to report any issues with equipment and view the current status of all equipment in real-time.
Process: Widget Corp’s development team decides to use TDD to ensure the quality and functionality of the new web part. Here’s how they approach it:
- Requirement Analysis:
- The web part must allow users to report new equipment issues.
- It should display a list of all equipment with their current status.
- Users should be able to filter the list based on the equipment’s status.
- Test Case Creation:
- Write a test to ensure that users can submit a new issue report.
- Write a test to verify that the equipment list displays correctly.
- Write a test to check the filter functionality.
- Development Cycle:
- Implement the minimum code required to pass the first test.
- Run the test suite to ensure the new code passes all tests.
- Refactor the code for better performance and readability.
- Repeat the cycle for the next test case.
- Implementation:
- Use React for the web part’s user interface.
- Utilize PnPjs for communication with the SharePoint list storing equipment data.
- Apply Jest and Enzyme for unit testing and mocking SharePoint context.
- Testing and Refinement:
- Continuously run tests after each development cycle.
- Refine the user interface based on feedback from the user experience team.
- Ensure the web part is responsive and accessible.
- Deployment:
- Integrate the web part into Widget Corp’s SharePoint site.
- Monitor the web part’s performance and user feedback.
- Make iterative improvements based on real-world usage.
Outcome: The “Equipment Status Tracker” web part is successfully deployed to Widget Corp’s SharePoint site. The TDD approach results in a reliable and user-friendly component that enhances the productivity of the employees. The development team is confident in the quality of their code, and future maintenance is simplified due to the comprehensive test suite created during development.
This use case illustrates how Widget Corp effectively applied TDD to their SPFx project, resulting in a robust and maintainable solution that meets the company’s needs.
Conclusion
Integrating TDD into your SPFx development workflow can significantly improve the quality and reliability of your SharePoint solutions. By writing tests first and coding second, you ensure that each component is thoroughly vetted and meets the project’s requirements. Embrace TDD, and watch your SPFx projects thrive in the hands of your users.