Microsoft Cloud Native and Aspire Innovation Collaboration
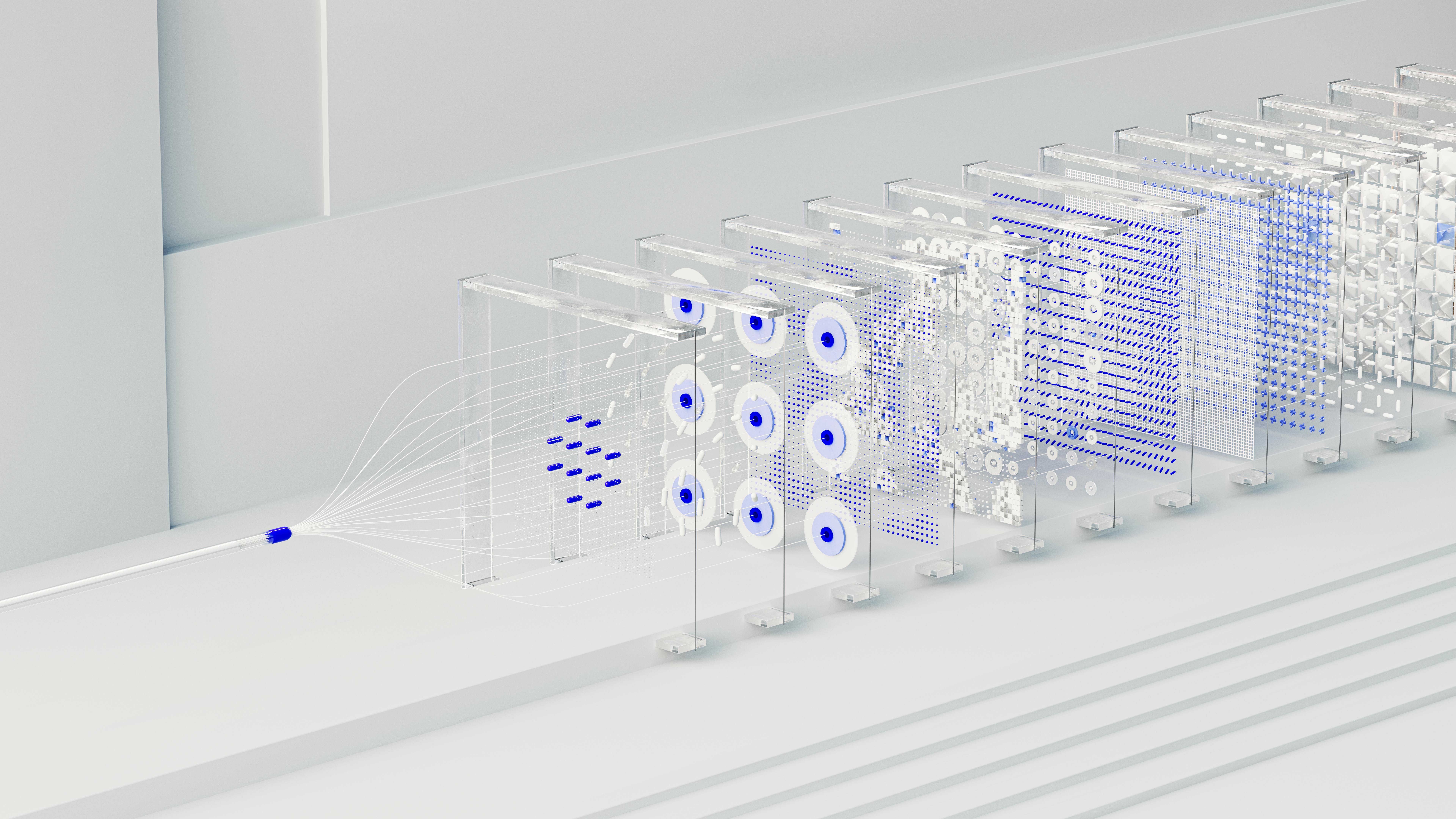
Microsoft Cloud Native
Cloud-native development is a modern approach to building and running applications that fully exploit the advantages of the cloud computing model. Microsoft has been at the forefront of this movement, providing tools and frameworks that enable developers to create scalable, resilient, and manageable applications.
Key Components of Microsoft Cloud Native
- .NET Aspire:
- Overview: .NET Aspire is an opinionated, cloud-ready stack designed for building observable, production-ready, distributed applications. It leverages a collection of NuGet packages that address specific cloud-native concerns.
- Orchestration: Enhances local development by simplifying the management of configuration and interconnections of cloud-native apps. It provides abstractions for service discovery, environment variables, and container configurations.
- Integrations: Offers standardized interfaces for commonly used services like Redis and Postgres, ensuring seamless connectivity with your app.
- Tooling: Comes with project templates and tooling experiences for Visual Studio, Visual Studio Code, and the dotnet CLI, making it easier to create and interact with .NET Aspire projects.
- Azure Kubernetes Service (AKS):
- Scalability: AKS allows developers to deploy, manage, and scale containerized applications using Kubernetes, providing a robust platform for cloud-native applications.
- Integration: Seamlessly integrates with other Azure services, enhancing the overall development and deployment experience.
- Azure Functions:
- Serverless Computing: Enables developers to build applications without worrying about infrastructure management. Azure Functions automatically scale and only charge for the compute resources used during execution.
Patterns and Practices
- Microservices Architecture:
- Description: Breaks down applications into smaller, independent services that can be developed, deployed, and scaled independently.
- Benefits: Enhances scalability, resilience, and agility.
- CI/CD Pipelines:
- Description: Continuous Integration and Continuous Deployment pipelines automate the process of building, testing, and deploying applications.
- Benefits: Reduces manual errors, provides faster feedback, and ensures consistent deployments.
- Infrastructure as Code (IaC):
- Description: Manages and provisions computing infrastructure through machine-readable definition files.
- Benefits: Ensures consistency, reduces configuration drift, and enables version control.
Technologies Used
- .NET Core: A cross-platform framework for building modern, cloud-based applications.
- Docker: A platform for developing, shipping, and running applications in containers.
- Kubernetes: An open-source system for automating the deployment, scaling, and management of containerized applications.
- Azure DevOps: A suite of tools for managing the entire application lifecycle, including CI/CD pipelines.
- Azure Monitor: Provides full-stack monitoring, advanced analytics, and intelligent insights.
Use Case: Contoso Retail
Contoso Retail is a fictional e-commerce company looking to modernize its platform using cloud-native technologies. The goal is to create a scalable, resilient, and manageable application that can handle high traffic during peak shopping seasons.
Architecture Overview
- Frontend: A React-based single-page application (SPA) hosted on Azure Static Web Apps.
- Backend: A set of microservices built with .NET Core, running on Azure Kubernetes Service (AKS).
- Database: Azure SQL Database for relational data and Azure Cosmos DB for NoSQL data.
- Authentication: Azure Active Directory B2C for customer authentication.
- Monitoring: Azure Monitor and Application Insights for monitoring and diagnostics.
Advantages
- Scalability: The use of AKS allows Contoso Retail to scale its services up or down based on demand, ensuring optimal performance during peak times.
- Resilience: Microservices architecture ensures that failures in one service do not affect the entire application, enhancing overall system resilience.
- Manageability: Azure DevOps and Infrastructure as Code (IaC) practices streamline the deployment and management of the application, reducing manual errors and configuration drift.
- Cost Efficiency: Azure Functions and serverless computing models ensure that Contoso Retail only pays for the compute resources used, optimizing costs.
- Security: Azure Active Directory B2C provides robust authentication and authorization mechanisms, ensuring secure access to the application.

Use Case for Coding and Governance
Scenario: Contoso Retail wants to implement a new feature that allows customers to view personalized product recommendations based on their browsing history.
- Microservice Implementation: Recommendation Service
C#
using Microsoft.AspNetCore.Mvc; using Microsoft.Extensions.Logging; using System.Collections.Generic; using System.Threading.Tasks; namespace ContosoRetail.RecommendationService.Controllers { [ApiController] [Route("[controller]")] public class RecommendationsController : ControllerBase { private readonly ILogger<RecommendationsController> _logger; public RecommendationsController(ILogger<RecommendationsController> logger) { _logger = logger; } [HttpGet("{customerId}")] public async Task<IEnumerable<Product>> GetRecommendations(string customerId) { // Simulate fetching recommendations based on customer browsing history var recommendations = new List<Product> { new Product { Id = 1, Name = "Laptop", Price = 999.99M }, new Product { Id = 2, Name = "Smartphone", Price = 499.99M } }; return await Task.FromResult(recommendations); } } public class Product { public int Id { get; set; } public string Name { get; set; } public decimal Price { get; set; } } }
- CI/CD Pipeline for Recommendation Service: Azure DevOps YAML Pipeline
trigger: - main pool: vmImage: 'ubuntu-latest' steps: - task: UseDotNet@2 inputs: packageType: 'sdk' version: '5.x' installationPath: $(Agent.ToolsDirectory)/dotnet - script: dotnet build --configuration Release displayName: 'Build project' - script: dotnet test --configuration Release displayName: 'Run tests' - task: Docker@2 inputs: containerRegistry: '$(dockerRegistryServiceConnection)' repository: 'contosoretail/recommendationservice' command: 'buildAndPush' Dockerfile: '**/Dockerfile' tags: | $(Build.BuildId)
- Governance Practices
- Policy Enforcement: Use Azure Policy to enforce organizational standards and assess compliance at scale. For example, ensure that all resources are tagged appropriately and that only approved VM sizes are used.
- Security: Implement Azure Security Center to provide unified security management and advanced threat protection across hybrid cloud workloads.
- Monitoring and Logging: Use Azure Monitor and Application Insights to collect, analyze, and act on telemetry data from the application. Set up alerts for critical metrics and logs to proactively address issues.
- Cost Management: Use Azure Cost Management and Billing to monitor and control spending. Set budgets and alerts to ensure that the project stays within financial constraints.
By leveraging cloud-native technologies and best practices, Contoso Retail can build a robust, scalable, and secure e-commerce platform. The use of microservices, CI/CD pipelines, and governance practices ensures that the application is not only efficient but also manageable and compliant with organizational standards.
In the ever-evolving landscape of software development, cloud-native applications have become the cornerstone of modern, scalable, and resilient systems. Enter .NET Aspire, a groundbreaking technology stack designed to simplify and enhance the cloud-native development experience for .NET developers.
What is .NET Aspire?
.NET Aspire is an opinionated stack that leverages the powerful .NET Framework to streamline the development of cloud-native applications. It provides a curated set of tools and libraries that address common cloud-native concerns, such as orchestration, observability, and scalability, right out of the box.
Core Features of .NET Aspire
- Orchestration: .NET Aspire includes built-in orchestration support, allowing developers to manage and coordinate microservices seamlessly. This ensures that services can communicate effectively and handle failures gracefully.
- Components and Tooling: The stack comes with a rich set of components and tools designed to simplify the development process. From integrated development environments (IDEs) like Visual Studio and VS Code to the Azure Developer CLI (AZD), .NET Aspire provides everything you need to build, test, and deploy cloud-native applications efficiently.
- Observability: One of the key challenges in cloud-native development is maintaining visibility into the system’s health and performance. .NET Aspire addresses this with robust observability features, including logging, tracing, and metrics, enabling developers to monitor and troubleshoot their applications effectively.
- Azure Integration: .NET Aspire seamlessly integrates with Azure services, making it easy to deploy and manage applications in the cloud. The “azd up” experience, for instance, allows developers to move their locally-running apps to the cloud with minimal effort.
Getting Started with .NET Aspire
To get started with .NET Aspire, developers can use the .NET Aspire template in Visual Studio or VS Code. This template sets up a new project with all the necessary components and configurations, allowing you to focus on writing code rather than setting up infrastructure Here

Use Case: Contoso Retail – Cloud-Native E-commerce Platform
Scenario: Contoso Retail wants to modernize its e-commerce platform to improve scalability, resilience, and customer experience. They decide to leverage .NET Aspire for cloud-native development and deploy their solution on Azure.
Objectives:
- Scalability: Handle increased traffic during peak shopping seasons.
- Resilience: Ensure high availability and quick recovery from failures.
- Customer Experience: Provide a seamless and responsive shopping experience.
Architecture Overview
- Microservices: Break down the monolithic application into microservices (e.g., Product Catalog, Order Management, User Authentication).
- Containerization: Use Docker to containerize microservices.
- Orchestration: Deploy containers using Kubernetes (AKS – Azure Kubernetes Service).
- Observability: Implement logging, monitoring, and tracing using Azure Monitor and Application Insights.
- CI/CD: Set up continuous integration and deployment pipelines using Azure DevOps.
Code Samples
1. Product Catalog Microservice
Dockerfile:
FROM mcr.microsoft.com/dotnet/aspnet:6.0 AS base WORKDIR /app EXPOSE 80 FROM mcr.microsoft.com/dotnet/sdk:6.0 AS build WORKDIR /src COPY ["ProductCatalog/ProductCatalog.csproj", "ProductCatalog/"] RUN dotnet restore "ProductCatalog/ProductCatalog.csproj" COPY . . WORKDIR "/src/ProductCatalog" RUN dotnet build "ProductCatalog.csproj" -c Release -o /app/build FROM build AS publish RUN dotnet publish "ProductCatalog.csproj" -c Release -o /app/publish FROM base AS final WORKDIR /app COPY --from=publish /app/publish . ENTRYPOINT ["dotnet", "ProductCatalog.dll"]
Kubernetes Deployment:
apiVersion: apps/v1 kind: Deployment metadata: name: product-catalog spec: replicas: 3 selector: matchLabels: app: product-catalog template: metadata: labels: app: product-catalog spec: containers: - name: product-catalog image: contoso/product-catalog:latest ports: - containerPort: 80
2. CI/CD Pipeline (Azure DevOps)
azure-pipelines.yml:
trigger: - main pool: vmImage: 'ubuntu-latest' variables: dockerRegistryServiceConnection: 'ContosoRegistry' imageRepository: 'product-catalog' containerRegistry: 'contosoregistry.azurecr.io' tag: '$(Build.BuildId)' steps: - task: UseDotNet@2 inputs: packageType: 'sdk' version: '6.x' installationPath: $(Agent.ToolsDirectory)/dotnet - task: Docker@2 inputs: command: 'buildAndPush' repository: '$(containerRegistry)/$(imageRepository)' dockerfile: '**/Dockerfile' tags: | $(tag)
Best Practices
- Microservices Design: Ensure each microservice has a single responsibility and communicates with others via well-defined APIs.
- Containerization: Use lightweight, immutable containers to ensure consistency across environments.
- Orchestration: Use Kubernetes for automated deployment, scaling, and management of containerized applications.
- Observability: Implement comprehensive logging, monitoring, and tracing to gain insights into application performance and issues.
- CI/CD: Automate the build, test, and deployment processes to ensure rapid and reliable delivery of updates.
Impact of Good Governance
1. Security:
- Implement role-based access control (RBAC) to restrict access to sensitive resources.
- Use managed identities to avoid hardcoding credentials in code.
2. Compliance:
- Ensure adherence to industry standards and regulations (e.g., GDPR, PCI-DSS).
- Regularly audit and review security policies and practices.
3. Cost Management:
- Monitor resource usage and optimize for cost efficiency.
- Use Azure Cost Management tools to track and manage expenses.
4. Reliability:
- Implement disaster recovery plans and regular backups.
- Use Azure’s built-in high availability and fault tolerance features.
5. Performance:
- Continuously monitor and optimize application performance.
- Use autoscaling to handle varying loads efficiently.
Good governance ensures that Contoso Retail’s cloud-native platform is secure, compliant, cost-effective, reliable, and performant, ultimately leading to a better customer experience and business success.
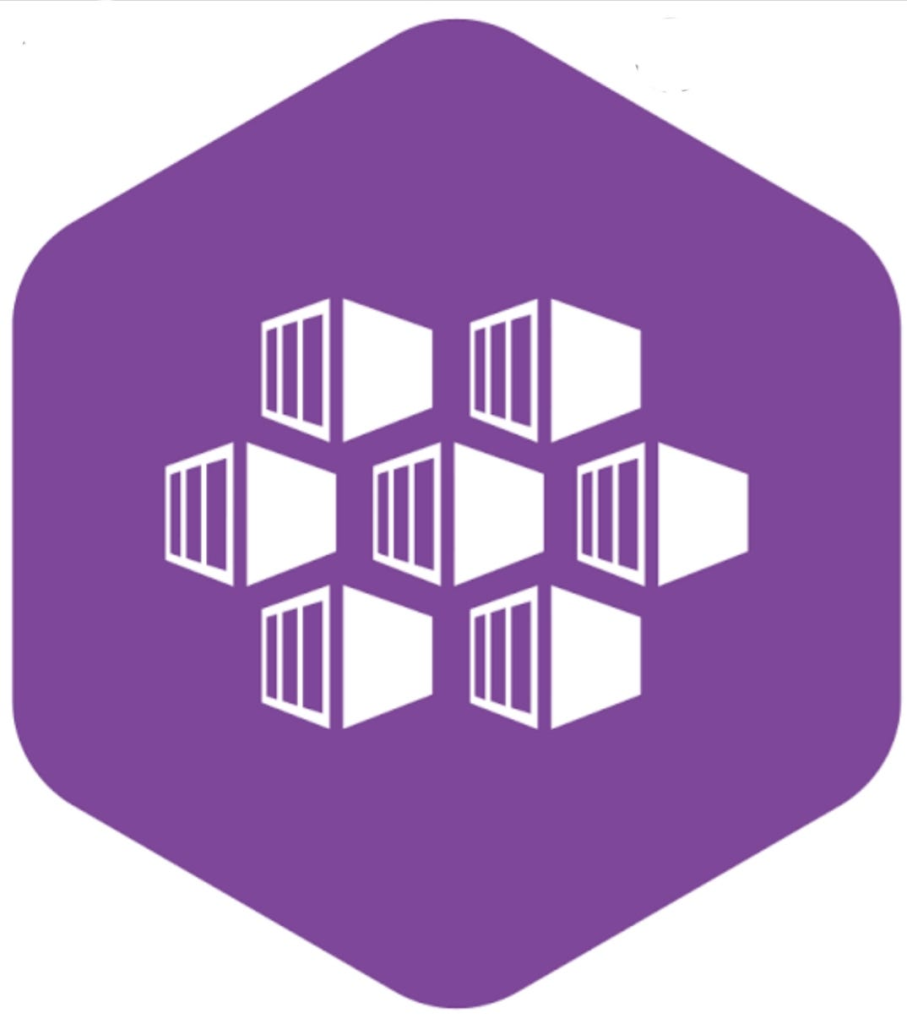
When to Use AKS
- Microservices Architecture:
- If your application is built using a microservices architecture, AKS can help manage and orchestrate these services efficiently. It provides features like automatic scaling, self-healing, and load balancing.
- Containerized Applications:
- When your applications are containerized using Docker, AKS offers a managed Kubernetes environment to deploy, manage, and scale these containers seamlessly.
- DevOps Integration:
- AKS integrates well with Azure DevOps, enabling continuous integration and continuous deployment (CI/CD) pipelines. This makes it easier to automate the build, test, and deployment processes.
- High Availability and Scalability:
- For applications that require high availability and need to scale dynamically based on demand, AKS provides robust scaling options and ensures minimal downtime.
- Managed Kubernetes:
- Hybrid and Multi-Cloud Deployments:
When Not to Use AKS
- Simple Applications:
- For simple applications that do not require the advanced features of Kubernetes, using AKS might be overkill. Azure App Service or Azure Container Instances (ACI) could be more appropriate for straightforward deployments.
- Non-Containerized Workloads:
- If your applications are not containerized, using AKS would require additional effort to containerize them first. In such cases, other Azure services like Virtual Machines or App Service might be more suitable.
- Resource Constraints:
- Running a Kubernetes cluster can be resource-intensive. If you have limited resources or budget, managing a full AKS cluster might not be cost-effective. ACI or Azure Functions could be more economical alternatives.
- Learning Curve:
- Kubernetes has a steep learning curve. If your team lacks experience with Kubernetes, there might be a significant investment in training and learning. For smaller teams or projects, simpler orchestration tools might be preferable.
- Specific Compliance Requirements:
- If your application has specific compliance or regulatory requirements that are not fully supported by AKS, you might need to consider other options or additional configurations to meet those requirements.
AKS is a powerful tool for managing containerized applications, especially in complex, scalable, and high-availability scenarios. However, it’s essential to evaluate your specific needs, resources, and expertise before deciding to use AKS. For simpler applications or non-containerized workloads, other Azure services might be more appropriate and cost-effective.
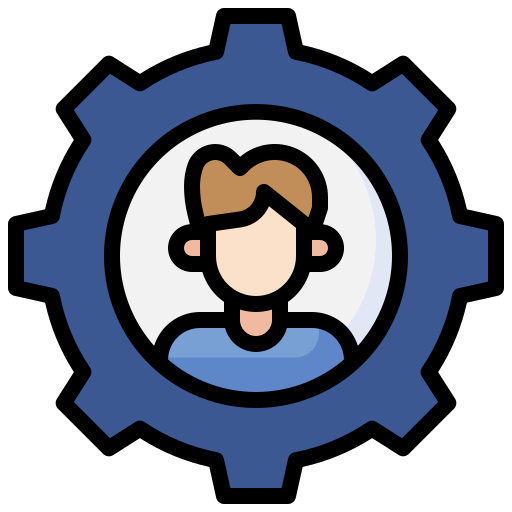
ROLES
1. Client Applications
Role:
- These are the interfaces through which users interact with the system. They can be web applications, mobile apps, or other client interfaces.
- They send requests to the backend services via the API Gateway.
2. API Gateway
Role:
- Acts as a single entry point for all client requests.
- Routes requests to the appropriate microservices.
- Provides functionalities like load balancing, authentication, rate limiting, and caching.
3. Microservices
Role:
- Product Catalog Service: Manages product information, including details, pricing, and availability.
- Order Management Service: Handles order processing, including order creation, payment processing, and order tracking.
- User Authentication Service: Manages user authentication and authorization, ensuring secure access to the system.
4. Container Registry
Role:
- Stores Docker images for the microservices.
- Ensures that the correct versions of images are used during deployment.
- Facilitates the sharing and reuse of container images across different environments.
5. Azure Kubernetes Service (AKS)
Role:
- Orchestrates and manages the deployment of containerized microservices.
- Provides features like automatic scaling, self-healing, and load balancing.
- Simplifies the management of Kubernetes clusters by handling the control plane operations.
6. Database
Role:
- Stores application data persistently.
- Azure SQL Database or Cosmos DB: Provides scalable, reliable, and secure data storage solutions.
- Ensures data consistency and availability across different microservices.
7. Observability
Role:
- Azure Monitor and Application Insights: Provide comprehensive logging, monitoring, and tracing capabilities.
- Help in gaining insights into the system’s health, performance, and usage patterns.
- Facilitate troubleshooting and performance optimization.
8. CI/CD Pipeline
Role:
- Azure DevOps: Automates the build, test, and deployment processes.
- Ensures that code changes are continuously integrated and deployed to production.
- Reduces the time and effort required to deliver new features and updates.
Key Benefits of Each Component
- Client Applications: Enhance user experience by providing responsive and intuitive interfaces.
- API Gateway: Simplifies client interactions and improves security and performance.
- Microservices: Enable modular development, independent scaling, and easier maintenance.
- Container Registry: Ensures consistency and efficiency in deploying containerized applications.
- AKS: Provides robust orchestration and management of containerized workloads.
- Database: Ensures reliable and scalable data storage.
- Observability: Enhances system reliability and performance through comprehensive monitoring.
- CI/CD Pipeline: Streamlines the development and deployment processes, ensuring faster delivery of updates.
Conclusion
Microsoft Cloud Native and .NET Aspire stand out as pivotal tools for fostering innovation and collaboration. By leveraging the robust capabilities of cloud-native technologies and the comprehensive .NET Aspire stack, organizations can build scalable, resilient, and efficient applications that meet the demands of modern business.
Innovation: Microsoft Cloud Native and .NET Aspire empower developers to innovate with agility. The seamless integration with Azure services, coupled with advanced orchestration, observability, and automation features, enables teams to experiment, iterate, and deploy new solutions faster than ever before.
Collaboration: These technologies also enhance collaboration across development teams. With a unified set of tools and best practices, developers can work together more effectively, share insights, and maintain consistency across projects. The integration with Azure DevOps further streamlines the CI/CD process, ensuring that updates and improvements are delivered smoothly and reliably.
Governance: Good governance practices are integral to maximizing the benefits of these technologies. By implementing robust security measures, ensuring compliance, managing costs, and maintaining high performance and reliability, organizations can achieve sustainable growth and success.
In summary, Microsoft Cloud Native and .NET Aspire provide a powerful foundation for driving innovation and collaboration, enabling organizations to stay ahead in a competitive market and deliver exceptional value to their customers.